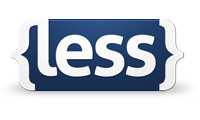
If you are like me, you have probably wondered why hitherto in CSS, we have not been able to use variables, functions and other general programming good practices given its verbose nature. Most developers that write CSS are familiar with programming principles and wouldn’t it be great if these could be applied to CSS? Well, we are in luck, say hello to LESS.
What is LESS?
LESS is a syntax or preprocessor that takes CSS and applies familiar programming principles such as variables, functions, mixins (more about this little number later) and nesting. What we get, is a smarter and dynamic language for writing CSS. You can take either the client-side or server-side approach when implementing LESS. When using the client-side approach, LESS is interpreted into regular CSS by way of JavaScript at runtime.
The ability to declare variables for example, gives us a more efficient way of changing values after the fact. For instance, if we decide that we now want to change a color used on the site from cyan to hot pink, we do not have to scour the stylesheet for all the instances of cyan. All we need to do is change it at source i.e where the variable is declared. LESS also brings the benefits of utility functions to CSS, a much welcome addition. LESS derives its name from yes, you guessed it; the ability to write less CSS.
How easy is it to get the ball rolling?
Compared to the other preprocessors, compass/SASS and Stylus, both of which need Ruby installed on your machine, LESS is child’s play so to speak, when it comes to implementation for client-side usage. It takes nothing but a couple of steps to get up and running.
Step 1
Create a .less file and link to it from the head
section like so: <link type=”text/css” rel=”stylesheet/less” href=”main.less” />
Step 2
For client-side usage, head off to lesscss.org, download less.js and reference it from the head
section.
<script src=”js/less.js”></script>
Important: It’s imperative that the stylesheet reference is placed above the Javascript script tag.
And that’s it. You are now ready to start writing LESS.
If you are however looking for some flexibility, you can configure certain aspects and functionality on the LESS global object. You can find out more about configuration in the documentation.
For the server-side approach, it is highly recommended that you use npm. Node.js (a server-side JavaScript interpreter) provides a package manager(npm) that runs through the command-line tool. This is the best way to install and use LESS server-side. I am not going to get into details on the command-line approach, but if you are interested, there is a very good wiki on the subject on github.
Now the syntax
The LESS syntax/language is very similar to CSS. All LESS and other preprocessors do is add cool features to CSS. By way of example, I will demonstrate some of these features.
Variables
LESS variables are prefixed with a @ sign and once declared can be referenced throughout the stylesheet. We can use variables for any CSS values e.g colors, text or numbers. This is an efficient way of storing values as we only have to change them in one place as opposed to regular CSS where one has to scan through the stylesheet for all instances of the value in question.
LESS
@bgColor:#c0ffee; @fontSize:12px; @borderStyle:dashed; @borderColor:#f0f; div{ width:150px; height:150px; background:@bgColor; border:1px @borderStyle @borderColor; }
here is the CSS after compilation:
COMPILED CSS
div{ width:150px; height:150px; background:#c0ffee; border:1px dashed #f0f; }
So, if we decide to change #c0ffee
to #bada55
, we only have to change the value of @bgColor
(declaration) and all the instances of @bgColor
within the stylesheet will be dynamically updated.
Mixins
Mixins are akin to functions in that they are blocks of code that can be invoked anywhere within the stylesheet. They can accept arguments similar to functions in other programming languages – say, JavaScript. A very good use case scenario is vendor prefixes. In many cases we find ourselves repeating vendor prefixes for features that are not yet supported in some browsers.
Using a mixin can make this more dynamic and efficient as I demonstrate below.
LESS
.border-radius(values){ -webkit-border-radius:$values; -moz-border-radius:$values; border-radius:$values; } div { .border-radius(10px); }
Now whenever we want to add rounded corners to an element, we just call our new border radius mixin and pass in a value. Here is the *compiled CSS;
COMPILED CSS
div{ -webkit-border-radius:10px; -moz-border-radius:10px; border-radius:10px; }
Imagine what we can do with this feature! For instance you could create a mixin with default values or pass your desired value at invocation. Here is another example;
//create a mixin that accepts an optional background color
.flag(@bgColor:”#cf0″){ background-color:@bgColor }
//apply styles from the mixin above
.highlight { .flag(); border:1px solid red; }
//or pass in a different color
.highlight2 { .flag(‘#333’); }
Color functions
Color functions are some of the built-in functions that come with LESS. They give us the ability to create variations of a single color. A good use case scenario is when you want to create gradients or hover colors. In the snippet below, I dynamically generate a link hover color using the darker color function;
LESS color function
@color:#c0ffee; a { color:@color; } a:hover { color:darken($color, 25%); //the hover link will compile to 25% darker than @color }
Other color functions include;
- lighten – lightens @color by a specified percentage
- saturate – compiles to a more saturated color
- desaturate – does the opposite of saturate
- mix – returns a mix of two colors
LESS has many more built functions that can do much more. Refer to the LESS documentation for more.
Nesting
If you are familiar with the CSS cascading principle, you will know that it leads to a lot of repetition. Say for example;
#main { color:#f0f; }
#main .first { color:#c0ffee; }
#main a { text-decoration:none; }
#main a:hover { tex-decoration:underline; }
LESS alleviates this repetitiveness by introducing nesting. We can re-write the above code as follows;
Nesting in LESS
#main { color:#f0f; .first{ color:#c0ffee; } a{ text-decoration:none; &:hover{ text-decoration:underline; } } }
Note the use of the ampasand(&) for pseudo classes. The same applies for element type selectors e.g p.warning
Operations
Yes, you read right. In LESS you can perform operations on variables and color values. Lets do some simple operations in LESS;
LESS Operations
@width: 150px; @height: 50px; div { width:(@width + 5); //this will evaluate to 155px height:(@height * 2); //this will evaluate to 100px }
Summary
LESS is picking up traction in the web development community primarily because, by adding useful features such as variables and functions, LESS saves time. Try it in your next project and you will not be disappointed. As I mentioned earlier, Compass/SASS and Stylus are the other CSS preprocessors at our disposal but I think LESS is by the far the easiest to work with.
A word of caution: Compiling client-side has a performance overhead, especially on big projects. It’s recommended that you either use caching on compile server-side.
Note: I should point out that I have used the term ‘compile’ loosely for emphasis. Strictly speaking JavaScript does not compile, it interprets.
Useful resources
- lesscss.org
- Introduction to Compass/SASS
- Elements.less – a set of ready mixins that augment LESS .
- preboot.less – – a collection of very handy mixins include grids.