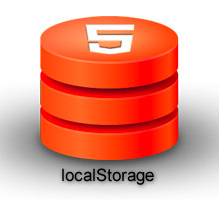
Here is a scenario that we can all relate to; You are filling in a long form and half way through, you inadvertently close the tab/window or worse still your laptop decides to crash. ‘Argh!, I was half way through that form, now I have to start again?’ comes to mind. Well, fret no more. Thanks to the localStorage API we can store form values (and more) on the fly so that in the event that the above happens, the values already entered will still be in browser memory. Storing persisting data has never been easier, and it lends itself well to enhancing user experience. I can here you say, but isn’t that a cookie? No way Jose! Sit back, relax and enjoy the ride!
What is this localStorage API thingy?
Simply put, localStorage
is a JS API (commonly referred to as part of the HTML5 spec) that facilitates data storage in the browser. This is in form of key/value pairs that persist even when the user navigates away from the page. Persistence is where the similarity between localStorage
and cookies ends. Not to be presumptuous, key – A name for a value to be stored. value – The value stored. Unlike Cookies,
- localStorage does not expire, Cookies do
- the localStorage API recommends up to 5MB, Cookies are small(approx. 4kb)
- The localStorage API is very easy to work with as it’s simply a JavaScript object made up of key/value pairs, Cookies are messy NOTE: even if an object, the localStorage object can only store simple data types i.e strings, numbers and floating points.
- localStorage is not sent in HTTP requests, Cookies are. This adds an overhead to your web application as unnecessary requests are made to the server.
localStorage
can be accessed via the global window
object and is supported in the latest iterations of all the major browsers, IE included. As a matter of fact localStorage
is supported as of IE8. To test for localStorage support, simply let Modernizr (you should be using it) do the detection for you, here is how;
if(Modernizr.localstorage){
//browser supports localStorage
}else{
//browser does NOT support localStorage
}
You however do not have to use Modernizr to test for localStorage support. You can simply check if the window
object has the localStorage property;
if(window.localStorage){
//browser supports localStorage
}else{
//browser does NOT support localStorage
}
but then again, Modernizr
aids the detection of all the other cool progressive enhancement features, so why not take advantage.
Crash course over!
So how do I use this nice API to save form data?
As I alluded to above, the localStorage API makes working with persistent data a breeze. It’s also very easy to implement. So without further ado, here we go;
localStorage implementation
if(Modernizr.localstorage){ //browser supports localstorage $(function(){ //lets store input field data in a field on blur. $('form').on('blur', 'input', function(){ //set a flag to indicate that we have saved data! localStorage.setItem('flag','formData'); //lets serialize the form data using jQuery's serializeArray() var data = $('form').serializeArray(); //lets loop through the data and save the input data $.each(data, function(i, obj){ localStorage.setItem(obj.name, obj.value); }); }); /*-------------------------------------------------------------- | if saved form data exists set input field values. | Note: this will naturally run before the above code | which is only triggered on the blur event. --------------------------------------------------------------*/ if (localStorage.getItem('flag')){ var data = $('form').serializeArray(); $.each(data, function(i, obj){ $("[name='"+obj.name+"']").val(localStorage.getItem(obj.name)); }); } }); }else{ //NO native localStorage support - resort to a fallback eg $.localStorage() }
In the above snippet, we test for localStorage
support which if successful, and after the DOM is ready, we use the blur event to trigger localStorage
, calling the setItem() method in particular. This little number will create key (obj.name), value (obj.value) pairs for all input fields other than the submit button and store these as an array of objects stored in browser memory. We do this by iterating through the serialized form data. In layman talk, every time the user moves away from a field we tell the browser to store the values in the input fields.
Great! We have now stored the data, but how do we then retrieve this data? Before we retrieve the data and populate the respective fields, note that we set a flag if localStorage.setItem() has been invoked on the form data. We can then maybe alert the user that form data was saved last time they filled in the form, but we can also use this flag as a test for presence of saved data. We then serialize the form data so that we can loop through it and set the values of the input fields. Note that instead of invoking the setItem() method, we are now calling upon its sibling getItem().VoilĂ ! Easy as pie.
Being the nice developers that we are, we can also provide a way for the user to clear the form fields if they so wish, by simply adding a button to clear input data. The click event handler for the button will be something akin to;
$(‘#clear-btn’).on(‘click’, function(){ localStorage.setItem(‘flag’,”) })
On clicking the ‘clear button’ the value of the flag will be set to empty string which is a falsey value. Consequently, when the user reloads the page, the code that sets the input values to the stored data will not execute/run.
Browser support
Summary
The fact that the localStorage
interface is a lot easier to use compared to the now ageing cookies, is the reason this API has had rapid adoption by many in the development community. There are many applications for localStorage
but I have used persisting form data as a way of whetting your appetite and I implore you to explore this cool API further. Hopefully, this will make storing and working with client-side data a tad bit easier.
That said, localStorage
should be used judiciously and users should always be made aware that their data is being stored albeit to improve user experience. Don’t forget those disclaimers!
Useful resources
- The past, present, and future of local storage– by Mark Pilgrim
- jQuery localStorage
- comply with EU cookie law – by Andi Smith
Good post Samson. I will definitely use localStorage more in my projects. BTW I like the custom font.
Ooh sounds great and I can see it being very useful . Thanks for this Samson.